How to Whitelist IP Addresses that Invoke Your REST API in AWS CDK
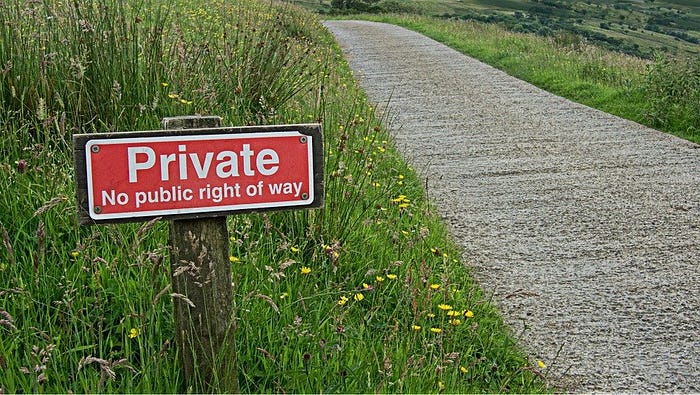
Whitelisting IPs means granting access to specific IP addresses. This can be useful when you are using a third-party service that will ping your REST API. If the third-party provides specific IP addresses, which most of them will for security purposes, it is important that you restrict calls to your API. An example could be a payment processor sending webhooks about recent billing changes etc.
First create your REST API with lambda integration. This will integrate an AWS Lambda function to an API Gateway method. We will need to add a policy statement that will deny all IPs that are not our specified whitelisted IPs. We’ll call that policy “testApiResourcePolicy” for now and will create it in the next step.
You can use the AWS CDK docs to further customize your REST API.
new apigw.LambdaRestApi(this, 'testApi', {
handler: lambdaFunction, // Input your lambda function
restApiName: 'test',
description: 'Test for Whitelisting IPs',
policy: testApiResourcePolicy, // We will implement this next
})
Now that we have our REST API created we will make the policy statement that will allow invocations to our REST API, but deny any IPs that are not our whitelisted addresses.
const testApiResourcePolicy = new iam.PolicyDocument({
statements: [
new iam.PolicyStatement({
actions: ['execute-api:Invoke'],
principals: [new iam.AnyPrincipal()],
resources: ['execute-api:/*/*/*'],
}),
new iam.PolicyStatement({
effect: iam.Effect.DENY,
principals: [new iam.AnyPrincipal()],
actions: ['execute-api:Invoke'],
resources: ['execute-api:/*/*/*'],
conditions: {
NotIpAddress: {
'aws:SourceIp': ['12.345.67.89', '98.765.43.21'],
},
},
}),
],
})
And that’s it, pretty simple! You can take a look at the AWS CDK docs to personalize your Policy Statement with the requirements you need.